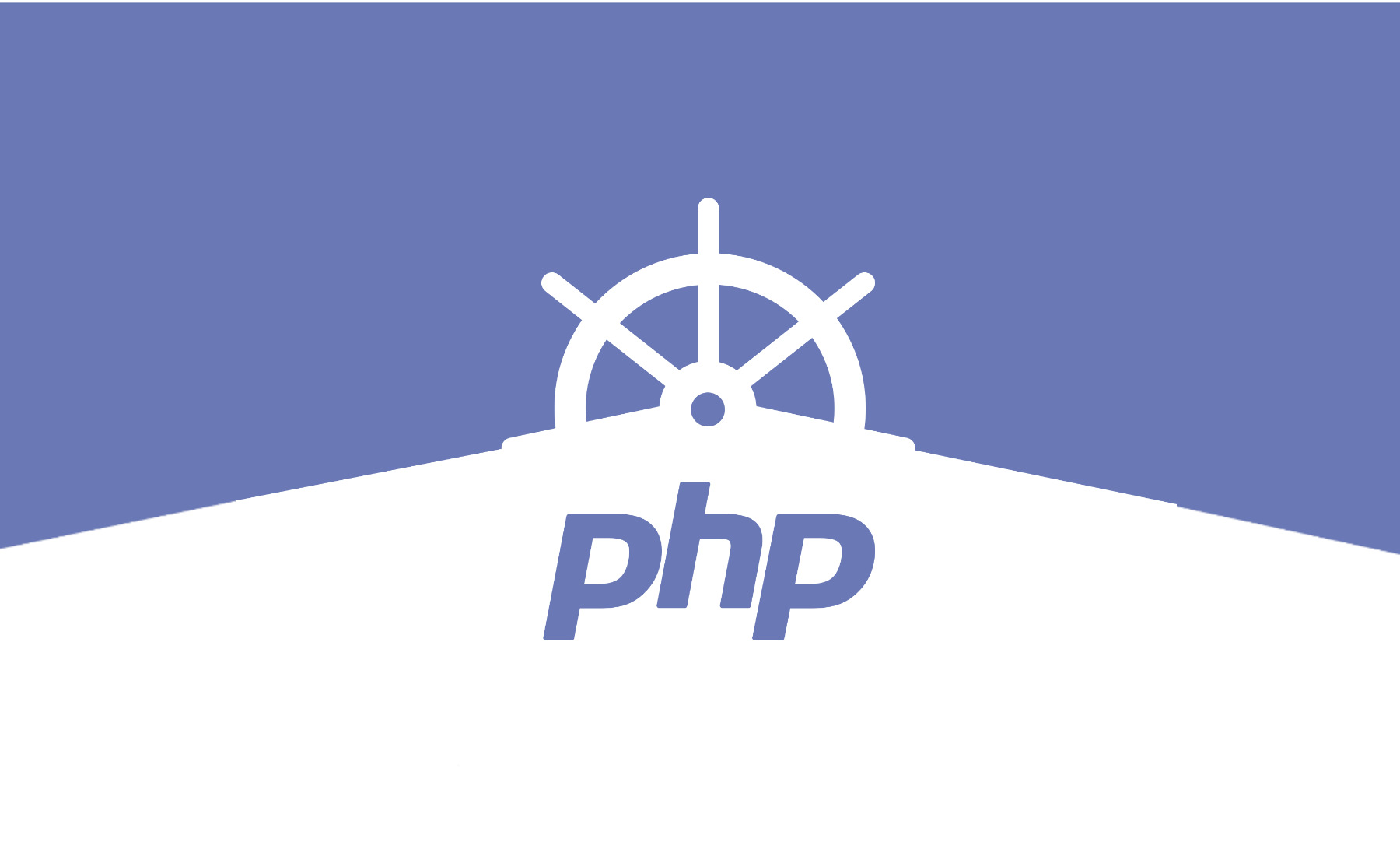
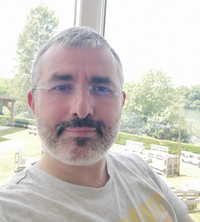
Gaël Trebos
First, you will need to have a Kubernetes cluster set up and running. If you don't have one already, you can use a tool like minikube to set up a local cluster for testing and development.
Once you have a cluster running, you can create a Kubernetes deployment to manage the PHP 8.3 pods and the Symfony application. The deployment will define the number of replicas, the container image to use, and any necessary environment variables or config maps.
Here's an example of a Kubernetes deployment YAML file for a PHP 8.3 and Symfony application:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-php-app
spec:
replicas: 3
selector:
matchLabels:
app: my-php-app
template:
metadata:
labels:
app: my-php-app
spec:
containers:
- name: my-php-app
image: my-php-image:8.3
ports:
- containerPort: 8000
env:
- name: SYMFONY_ENV
value: prod
- name: DATABASE_URL
# Please note that this is a simple tutorial, you should never put real DB details in clear in configs, use kube secrets to manage it
value: "mysql://user:password@database:3306/dbname"
volumeMounts:
- name: config-volume
mountPath: /app/config
volumes:
- name: config-volume
configMap:
name: my-php-app-config
Use Kube secrets !
Please note that this is a simple tutorial, you should never put real DB details in clear in configs, use kube secrets to manage it. Have a look to this if you want to know more about Kube's secrets.
This deployment will create 3 replicas of a container based on the "my-php-image:8.3" image, and expose port 8000. It also sets the SYMFONY_ENV and DATABASE_URL environment variables, and mounts a configMap named "my-php-app-config" to the "/app/config" path.
You can also create a Service to expose your application to the network, and a ConfigMap to store your application configuration.
apiVersion: v1
kind: Service
metadata:
name: my-php-app
spec:
selector:
app: my-php-app
ports:
- name: http
port: 80
targetPort: 8000
type: LoadBalancer
---
apiVersion: v1
kind: ConfigMap
metadata:
name: my-php-app-config
data:
config.yml: |
framework:
secret: "mysecretkey"
This service will allow external access to port 80, and route the traffic to port 8000 of the pods created by the deployment. And the configmap will store the configuration of the application in a file named config.yml.
After you have created these resources, you can use the kubectl
command-line tool to deploy and manage your application on the cluster.
To deploy the application, you can use the kubectl apply -f
command, passing in the path to the deployment YAML file
Once you have your application deployed and running on the Kubernetes cluster, you will need to set up an ingress to allow external traffic to reach your application. An ingress is a Kubernetes resource that allows you to configure how external traffic is routed to your application pods.
Here's an example of an ingress configuration for the PHP 8.3 and Symfony application:
apiVersion: networking.k8s.io/v1
kind: Ingress
metadata:
name: my-php-app
annotations:
nginx.ingress.kubernetes.io/rewrite-target: /
spec:
rules:
- host: example.com
http:
paths:
- path: /my-php-app
pathType: Prefix
pathRewrite: /
pathRedirect: /
backend:
service:
name: web
port:
number: 8080
Once you have created the ingress resource, you can use the kubectl apply -f
command, passing in the path to the ingress YAML file, to create the ingress on your cluster.
After the ingress is created, it may take some time for the changes to take effect and external traffic to be routed to your application pods. You can use the kubectl get ingress
command to check the status of the ingress and see if it has been assigned an external IP address.
Once the ingress is configured and working, you should be able to access your application by visiting "example.com/my-php-app" in a web browser.
You also need to make sure that your DNS is configured to point to the IP of your ingress controller(s) or to the load balancer that front your ingress controllers.
And you can use Kubernetes tools like Prometheus and Grafana to monitor the health of your application and its resources, and to troubleshoot any issues that may arise.
It is also important to keep in mind that this is just a basic configuration example, and that there are many other options and features available in Kubernetes for deploying and managing your application.
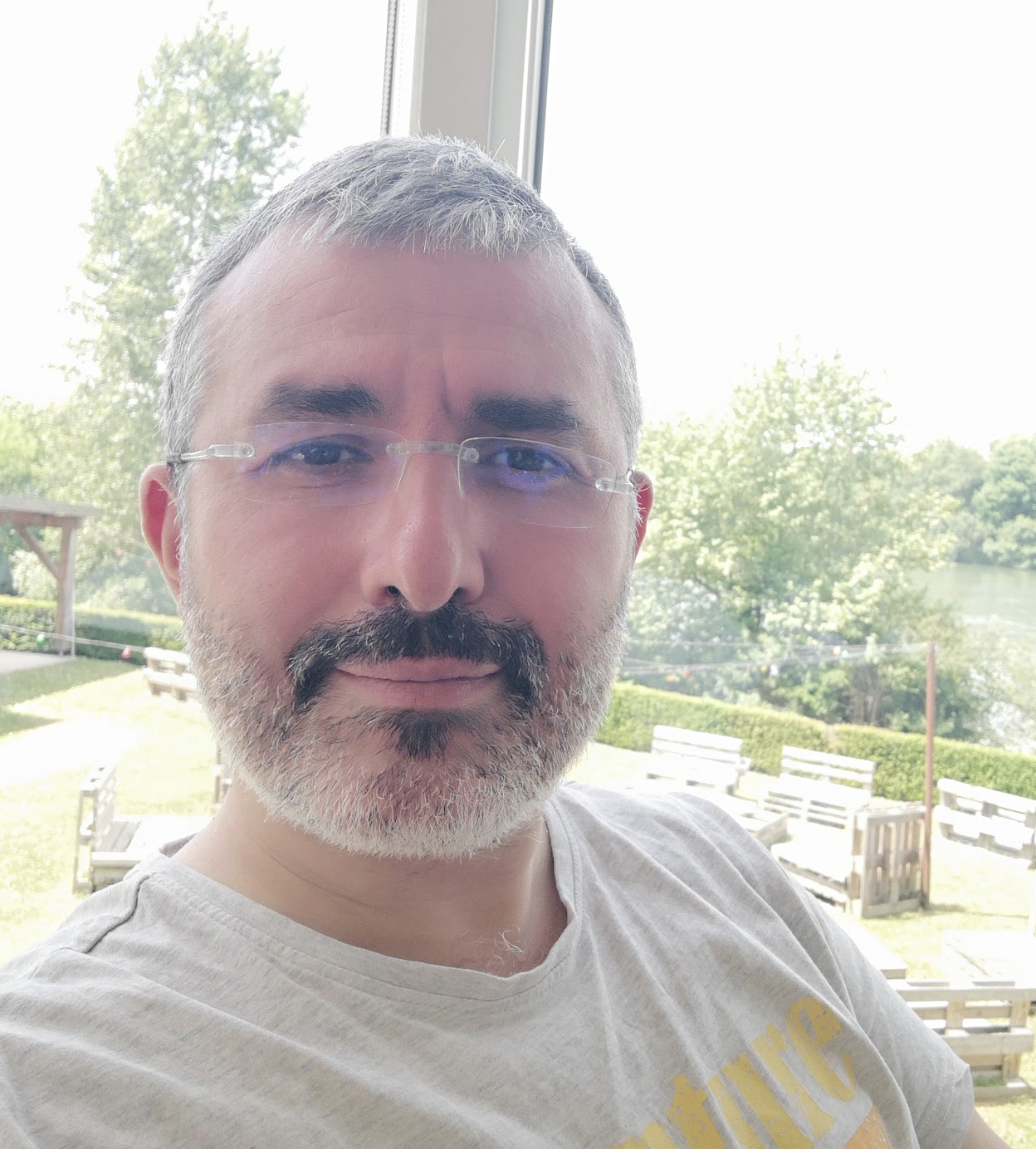
Redouane is co-Leading the Platform team, his role at Future is to build, maintain and expand our Vanilla Platform.
His main focuses are on the performance and High scalability of the architectures.
- Gaël TrebosTech Lead